Common UI Components
Introduction
Description
In game development, we often need to use some common UI components, such as buttons, input fields, scrolling lists, etc. If we were to redevelop these basic components each time, it would not only waste development time but also lead to bugs. Open-source common components allow developers to directly use these components to quickly build game interfaces, greatly improving development efficiency. These components have undergone performance optimization and stability testing and can be reliably used in production environments.
Basic Usage
UI File Structure
Generated script files will be in the following directories:
Scripts
├── UI
│ ├── HotfixView
│ │ └── UIBehaviour
│ │ └── CommonUI
│ └── ModelView
│ └── UIBehaviour
│ └── CommonUI
└── ...
Prefab directory structure corresponds to the following directories:
Assets
├── Bundles
│ └── UI
│ ├── Common // Common UI components
│ ├── Item // Loop list item prefabs
│ ├── Dlg // UI interface prefabs
│ └── Other // Other UI resources
└── ...
- Common: Stores common UI components, such as buttons, input fields, and other reusable UI components
- Item: Stores list item prefabs used in loop lists
- Dlg: Stores complete UI interface prefabs, such as main interface, inventory interface, etc.
- Other: Stores other UI-related resources, for example:
red dot prefabs
Common UI Component Code Generation Demonstration
Video Tutorial
The following video demonstrates the process of generating common UI component code using the UI framework
Notes
- The root node of a common component must start with
ES_
to be recognized and have code generated. Other nodes are named normally withE_
orEG_
.
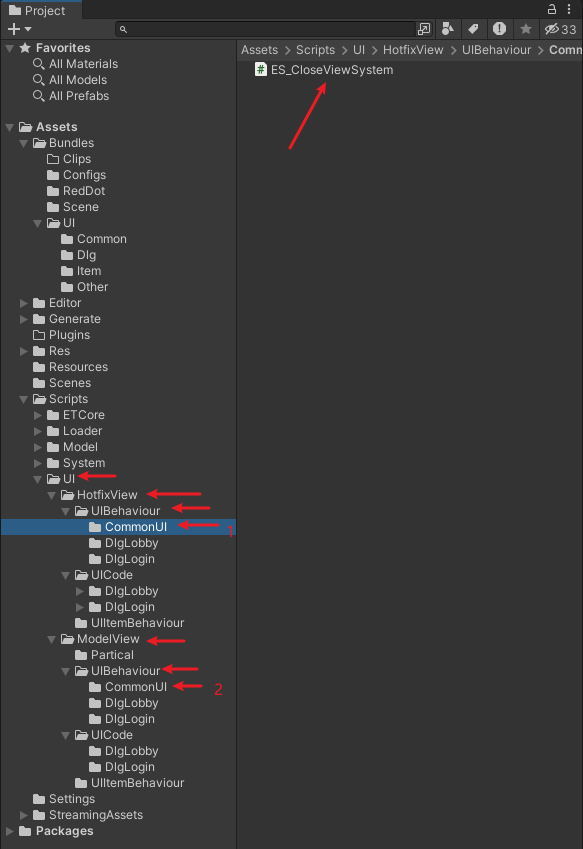
INFO
For development, we only need to focus on the files in the 2nd
folder highlighted in the image. After generation, the directory will look like this:
UI
├── HotfixView
│ └── UIBehaviour
│ └── CommonUI
│ └── ES_CloseViewSystem.cs
└── ...
ES_CloseViewSystem.cs
using UnityEngine;
using UnityEngine.UI;
namespace MH
{
[EntitySystem]
public class ES_CloseAwakeSystem : AwakeSystem<ES_Close, Transform>
{
protected override void Awake(ES_Close self, Transform transform)
{
self.uiTransform = transform;
}
}
[EntitySystem]
public class ES_CloseDestroySystem : DestroySystem<ES_Close>
{
protected override void Destroy(ES_Close self)
{
self.DestroyWidget();
}
}
public static partial class ES_CloseViewSystem
{
}
}
Best Practices
Let's see how to write code in the script
using UnityEngine;
using UnityEngine.UI;
namespace MH
{
//Other Code...
public static partial class ES_CloseViewSystem
{
public static void CloseWindow(this ES_Close self, Scene scene, WindowID windowID)
{
self.E_CloseButton.AddListener(() =>
{
var uiComponent = scene.GetComponent<UIComponent>();
uiComponent.CloseWindow(windowID);
});
}
public static void HideWindow(this ES_Close self, Scene scene, WindowID windowID)
{
self.E_CloseButton.AddListener(() =>
{
var uiComponent = scene.GetComponent<UIComponent>();
uiComponent.HideWindow(windowID);
});
}
}
}
INFO
- By using
self.
, you can directly operate on components. Two methods have been written, with logic forclosing
andhiding
the interface, based on theScene
passed in. This makes it more universal since you might need to close interfaces from either theCurrentScene
or theRoot
.
Example Code
TIP
Now let's go back to DlgLoginSystem.cs
, because earlier we dragged this common close component
onto the DlgLogin
prefab but haven't used it yet.
using System.Collections;
using System.Collections.Generic;
using System;
using UnityEngine;
using UnityEngine.UI;
namespace MH
{
public static class DlgLoginSystem
{
public static void RegisterUIEvent(this DlgLogin self)
{
self.View.ES_Close.HideWindow(self.Scene, WindowID.WindowID_Login);
self.View.E_LoginButton.AddListener(self.Login);
}
public static void Login(this DlgLogin self)
{
var account = self.View.E_AccountInputField.text;
var password = self.View.E_PasswordInputField.text;
Debug.Log($"Account: {account}, Password: {password}");
self.Scene.GetComponent<UIComponent>().HideWindow(WindowID.WindowID_Login);
}
public static void ShowWindow(this DlgLogin self, Entity contextData = null)
{
self.Refresh();
}
public static void Refresh(this DlgLogin self)
{
}
}
}
TIP
Now we can call the common component's logical method using self.View.ES_Close.HideWindow(self.Scene, WindowID.WindowID_Login);
Common Issues
Notes
- Ensure the UI prefab structure follows the standards
Technical Support
Get Help
- 💬 Join QQ group for discussion
(ET Framework Group)
: 474643097 - ⭐ Follow the project on GitHub for the latest updates