UI Framework
Introduction
Description
The EUI framework is a UI framework based on Unity UGUI, providing a complete UI management solution. The main features include:
- Creating and destroying UI interfaces
- Showing and hiding UI interfaces
- UI interface hierarchy management
- UI interface lifecycle management
- UI interface data binding
Through these features, various UI interfaces in the game can be conveniently managed, improving development efficiency.
Core Features
- Standardized Design: Fully compliant with ET framework coding standards, separation of logic and display layers, clean and highly readable code
- Zero Script Attachment: UI prefabs require no MonoBehaviour scripts attached
- Lifecycle Management: Fully controllable UI interface lifecycle
- Automatic Code Generation: No need to manually create scripts, declare methods, variables, or drag objects for assignment, everything is automatically generated with one click
Basic Usage
UI File Structure
Generated script files will be in the following directories:
Scripts
├── UI
│ ├── HotfixView
│ │ ├── UIBehaviour
│ │ ├── UICode
│ │ └── UIItemBehaviour
│ └── ModelView
│ ├── UIBehaviour
│ ├── UICode
│ └── UIItemBehaviour
└── ...
Prefab directory structure corresponds to the following directories:
Assets
├── Bundles
│ └── UI
│ ├── Common // Common UI components
│ ├── Item // Loop list item prefabs
│ ├── Dlg // UI interface prefabs
│ └── Other // Other UI resources
└── ...
- Common: Stores common UI components, such as buttons, input fields, and other reusable UI components
- Item: Stores list item prefabs used in loop lists
- Dlg: Stores complete UI interface prefabs, such as main interface, inventory interface, etc.
- Other: Stores other UI-related resources, for example:
red dot prefabs
UI Interface Code Generation Demonstration
Video Tutorial
The following video demonstrates the process of generating UI code using the UI framework
Notes
- The interface root node must start with
Dlg_
to be recognized and have code generated - Other nodes that need interaction must start with
E_
to be recognized and have code generated - For nodes starting with
EG_
, components on the node will be ignored during code generation, only keeping the RectTransform component
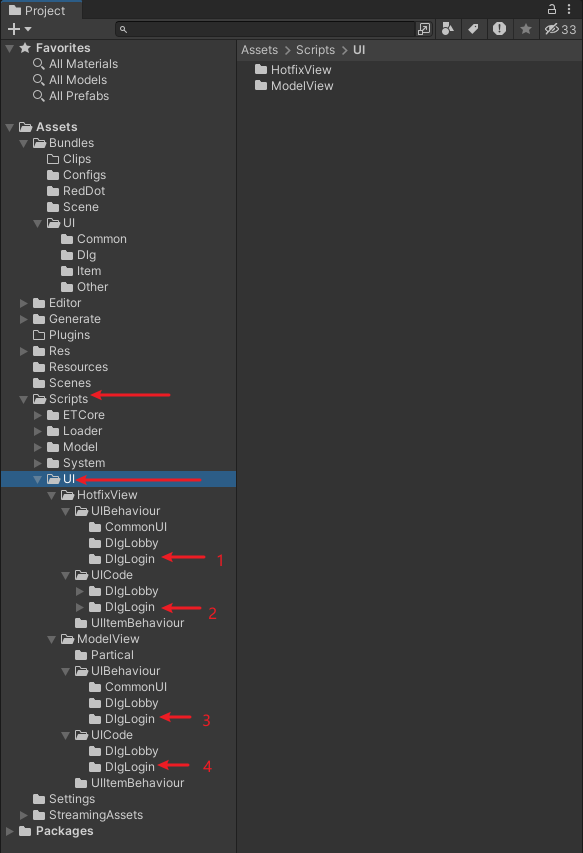
INFO
For development, we only need to focus on the files in the 2nd
folder highlighted in the image. After generation, the directory will look like this:
UI
├── HotfixView
│ └── UICode
│ └── DlgLogin
│ ├── Event
│ │ └── DlgLoginEventHandler.cs //Can set interface priority and lifecycle functions
│ └── DlgLoginSystem.cs //Main file for writing code
└── ...
DlgLoginEventHandler.cs
namespace MH
{
[AUIEvent(WindowID.WindowID_Login)]
public class DlgLoginEventHandler : IAUIEventHandler
{
public void OnInitWindowCoreData(UIBaseWindow uiBaseWindow)
{
uiBaseWindow.windowType = UIWindowType.Normal;
}
public void OnInitComponent(UIBaseWindow uiBaseWindow)
{
uiBaseWindow.AddComponent<DlgLogin>().AddComponent<DlgLoginViewComponent>();
}
public void OnRegisterUIEvent(UIBaseWindow uiBaseWindow)
{
uiBaseWindow.GetComponent<DlgLogin>().RegisterUIEvent();
}
public void OnShowWindow(UIBaseWindow uiBaseWindow, Entity contextData = null)
{
uiBaseWindow.GetComponent<DlgLogin>().ShowWindow(contextData);
}
public void OnHideWindow(UIBaseWindow uiBaseWindow)
{
}
public void BeforeUnload(UIBaseWindow uiBaseWindow)
{
}
}
}
DlgLoginSystem.cs
namespace MH
{
public static class DlgLoginSystem
{
public static void RegisterUIEvent(this DlgLogin self)
{
}
public static void ShowWindow(this DlgLogin self, Entity contextData = null)
{
}
}
}
The UI interface currently has 4 hierarchy levels:
namespace MH
{
public enum UIWindowType
{
Normal, // Normal main interface
Fixed, // Fixed window
PopUp, // Pop-up window
Other, // Other windows
}
}
Their corresponding Order in Layer in Unity nodes are: 0
, 10
, 20
, 30
.
Best Practices
Let's see how to write code in the script
namespace MH
{
public static class DlgLoginSystem
{
public static void RegisterUIEvent(this DlgLogin self)
{
self.View.E_LoginButton.AddListener(self.Login);
}
public static void Login(this DlgLogin self)
{
var account = self.View.E_AccountInputField.text;
var password = self.View.E_PasswordInputField.text;
Debug.Log($"Account: {account}, Password: {password}");
}
public static void ShowWindow(this DlgLogin self, Entity contextData = null)
{
self.Refresh();
}
public static void Refresh(this DlgLogin self)
{
}
}
}
INFO
- By using
self.View.
, you can directly operate on components. Register button events in theRegisterUIEvent
method, and write specific login logic in theLogin
method. ShowWindow
is a method that will be called once when the interface opens, where you can initialize the data on the page
Example Code
// Open UI
self.Root.GetComponent<UIComponent>().ShowWindow(WindowID.WindowID_Login);
// Hide UI
self.Root.GetComponent<UIComponent>().HideWindow(WindowID.WindowID_Login);
// Close UI
self.Root.GetComponent<UIComponent>().CloseWindow(WindowID.WindowID_Login);
// Get UI component
self.Root.GetComponent<UIComponent>().GetDlgLogic<DlgLogin>().Refresh();
INFO
- You can operate on UI by getting the
UIComponent
component on the entity.
Common Issues
Notes
- Ensure the UI prefab structure follows the standards
Technical Support
Get Help
- 💬 Join QQ group for discussion
(ET Framework Group)
: 474643097 - ⭐ Follow the project on GitHub for the latest updates