UI框架
简介
说明
EUI框架是一个基于Unity UGUI的UI框架,它提供了一套完整的UI管理方案。主要功能包括:
- UI界面的创建和销毁
- UI界面的显示和隐藏
- UI界面的层级管理
- UI界面的生命周期管理
- UI界面的数据绑定
通过这些功能,可以方便地管理游戏中的各种UI界面,提高开发效率。
核心特性
- 规范设计: 完全符合ET框架编码规范,逻辑层与显示层分离,代码简洁可阅读性强
- 零脚本挂载: UI预设物无需任何MonoBehaviour脚本挂载
- 生命周期管理: 完全自主可控的UI界面生命周期
- 自动代码生成: 无需手动创建脚本、声明方法、变量、拖拽物体赋值,全部一键自动生成
基础用法
UI文件结构
生成的脚本文件会对应在如下目录:
Scripts
├── UI
│ ├── HotfixView
│ │ ├── UIBehaviour
│ │ ├── UICode
│ │ └── UIItemBehaviour
│ └── ModelView
│ ├── UIBehaviour
│ ├── UICode
│ └── UIItemBehaviour
└── ...
预制体目录结构对应如下目录:
Assets
├── Bundles
│ └── UI
│ ├── Common // 通用UI组件
│ ├── Item // 循环列表项预制体
│ ├── Dlg // UI界面预制体
│ └── Other // 其他UI资源
└── ...
- Common: 存放通用UI组件,如按钮、输入框等可复用的UI组件
- Item: 存放循环列表中使用的列表项预制体
- Dlg: 存放完整的UI界面预制体,如主界面、背包界面等
- Other: 存放其他UI相关资源,例如:
红点预制体
构建UI界面代码使用演示
视频教程
以下视频展示了UI框架生成UI代码的使用流程
注意事项
- 界面根节点需要以
Dlg_
开头才会识别生成代码 - 其余需要交互的节点需要以
E_
开头才会识别生成代码 - 对于节点
EG_
开头的,在生成代码的时候会忽略节点身上的组件,只保留RectTransform组件
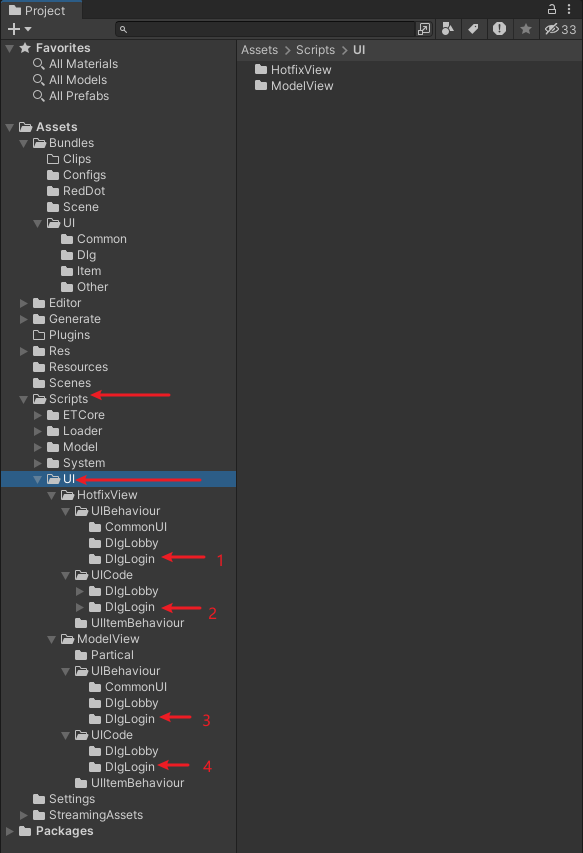
INFO
对于开发,我们只需要关注标注的第2个
文件夹下的文件,生成完成后该目录会是这样的:
UI
├── HotfixView
│ └── UICode
│ └── DlgLogin
│ ├── Event
│ │ └── DlgLoginEventHandler.cs //可以设置界面的优先级以及生命周期函数
│ └── DlgLoginSystem.cs //主要编写代码的文件
└── ...
DlgLoginEventHandler.cs
csharp
namespace MH
{
[AUIEvent(WindowID.WindowID_Login)]
public class DlgLoginEventHandler : IAUIEventHandler
{
public void OnInitWindowCoreData(UIBaseWindow uiBaseWindow)
{
uiBaseWindow.windowType = UIWindowType.Normal;
}
public void OnInitComponent(UIBaseWindow uiBaseWindow)
{
uiBaseWindow.AddComponent<DlgLogin>().AddComponent<DlgLoginViewComponent>();
}
public void OnRegisterUIEvent(UIBaseWindow uiBaseWindow)
{
uiBaseWindow.GetComponent<DlgLogin>().RegisterUIEvent();
}
public void OnShowWindow(UIBaseWindow uiBaseWindow, Entity contextData = null)
{
uiBaseWindow.GetComponent<DlgLogin>().ShowWindow(contextData);
}
public void OnHideWindow(UIBaseWindow uiBaseWindow)
{
}
public void BeforeUnload(UIBaseWindow uiBaseWindow)
{
}
}
}
DlgLoginSystem.cs
csharp
namespace MH
{
public static class DlgLoginSystem
{
public static void RegisterUIEvent(this DlgLogin self)
{
}
public static void ShowWindow(this DlgLogin self, Entity contextData = null)
{
}
}
}
UI界面目前设置了4个层级分别是:
csharp
namespace MH
{
public enum UIWindowType
{
Normal, // 普通主界面
Fixed, // 固定窗口
PopUp, // 弹出窗口
Other, //其他窗口
}
}
其在Unity节点中对应的Order in Layer分别是:0
、10
、20
、30
。
最佳实践
接下来看看如何在脚本里面编写其代码
csharp
namespace MH
{
public static class DlgLoginSystem
{
public static void RegisterUIEvent(this DlgLogin self)
{
self.View.E_LoginButton.AddListener(self.Login);
}
public static void Login(this DlgLogin self)
{
var account = self.View.E_AccountInputField.text;
var password = self.View.E_PasswordInputField.text;
Debug.Log($"账号:{account},密码:{password}");
}
public static void ShowWindow(this DlgLogin self, Entity contextData = null)
{
self.Refresh();
}
public static void Refresh(this DlgLogin self)
{
}
}
}
INFO
- 通过使用
self.View.
的方式来就可以直接对组件进行操作,在RegisterUIEvent
方法里面对按钮进行事件的注册,在Login
方法里编写具体的登录逻辑。 ShowWindow
是界面打开的时候会调用一次的方法,这里可以初始化一下页面上的数据
示例代码
csharp
// 打开UI
self.Root.GetComponent<UIComponent>().ShowWindow(WindowID.WindowID_Login);
// 隐藏UI
self.Root.GetComponent<UIComponent>().HideWindow(WindowID.WindowID_Login);
// 关闭UI
self.Root.GetComponent<UIComponent>().CloseWindow(WindowID.WindowID_Login);
// 获取UI组件
self.Root.GetComponent<UIComponent>().GetDlgLogic<DlgLogin>().Refresh();
INFO
- 通过获取实体身上的
UIComponent
组件的方式来就可以来操作UI。
常见问题
注意事项
- 确保UI预制体结构符合规范
技术支持
获取帮助
- 💬 加入QQ群讨论交流
(ET框架群)
: 474643097 - ⭐ 在GitHub上关注项目获取最新更新